TypeError: unhashable type: ‘dict’

TypeError: unhashable type: 'dict'
In Python, errors can occur when we try to perform operations that are incompatible with the data types being used. One common error is “TypeError: unhashable type: ‘dict'”, which arises when a dictionary (a mutable data type) is used in a context that requires a hashable (immutable) type. This error can be confusing for beginners, so understanding its cause and how to resolve it is essential for smoother programming.
What is the “TypeError: unhashable type: ‘dict'”?
This error is raised when you attempt to use a dictionary as a key in another dictionary or as an element in a set. Both dictionaries and sets require their keys or elements to be hashable. A hashable object in Python is an object that has a fixed hash value during its lifetime, meaning its contents cannot change. A dictionary, on the other hand, is mutable, meaning its contents can change, making it unhashable.
For example, the following code causes this error:
my_dict = {}
set_of_dicts = {my_dict} # Raises TypeError: unhashable type: 'dict'
Why Does This Happen?
Python uses hash values to efficiently compare keys in a dictionary and elements in a set. Since dictionaries can be modified (keys and values can be added, removed, or changed), they do not have a consistent hash value. As a result, Python prevents you from using them as keys in dictionaries or as elements in sets.
Read Also: Error: error:0308010c:digital envelope routines::unsupported
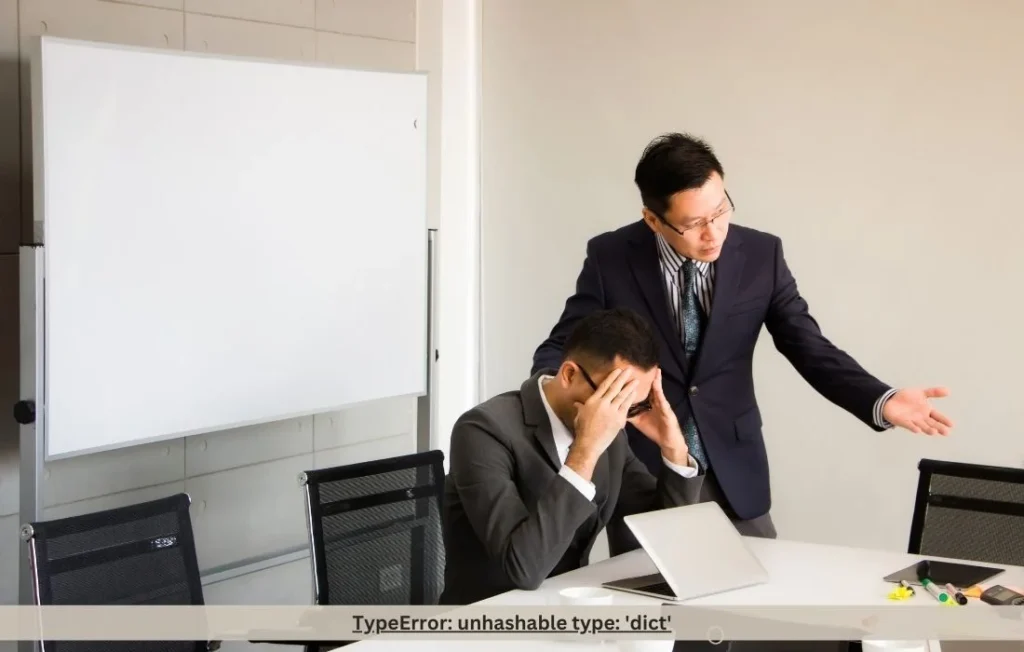
How to Resolve the Error?
There are several ways to fix this error, depending on what you’re trying to achieve.
- Convert the dictionary to an immutable type: If you want to use a dictionary-like structure as a key or set element, you can convert it to an immutable data type like a tuple:
my_dict = {'key1': 'value1', 'key2': 'value2'}
set_of_tuples = {tuple(my_dict.items())} # Works fine. TypeError: unhashable type: 'dict'
2. Use a frozenset
for dictionary keys: If you are specifically working with the dictionary’s keys and need them in a hashable format, you can convert them into a frozenset
(an immutable set):
my_dict = {'key1': 'value1', 'key2': 'value2'}
set_of_keys = {frozenset(my_dict.items())}. TypeError: unhashable type: 'dict'
3. Avoid using dictionaries in sets or as dictionary keys: In cases where dictionaries are not the appropriate data structure, consider using hashable objects like strings, tuples, or integers.
FAQs
1. What does the error “TypeError: unhashable type: ‘dict'” mean?
This error means that a dictionary, which is a mutable object, is being used in a context that requires a hashable (immutable) object. This usually happens when trying to use a dictionary as a key in another dictionary or as an element in a set.
2. Why can’t dictionaries be used as keys in a dictionary or as elements in a set?
Dictionaries are mutable, meaning their content can change over time. Since both sets and dictionary keys require elements to have a consistent hash value (which mutable objects don’t have), dictionaries cannot be used in these contexts.
3. How can I make a dictionary hashable?
You can convert a dictionary into an immutable data structure such as a tuple or frozenset. For example:my_dict = {'key1': 'value1'}
immutable_version = tuple(my_dict.items()) # Using tuple . TypeError: unhashable type: 'dict'
4. Can I use a list or a set as a dictionary key?
No, lists and sets are also mutable types, so they cannot be used as keys in a dictionary for the same reason that dictionaries cannot. Instead, use tuples or frozensets for immutable, hashable versions of those collections.
5. How do I fix “TypeError: unhashable type: ‘dict'” in my code?
It depends on what you’re trying to do:
If you’re trying to use a dictionary as a key or set element, consider converting it to an immutable type like a tuple or frozenset.
If dictionaries are not needed in this context, consider using other hashable data types like strings, numbers, or tuples.
6. When would I need to convert a dictionary to a tuple or frozenset?
You would convert a dictionary to a tuple or frozenset when you need to maintain its data in a format that can be used as an element in a set or as a key in a dictionary. For example, when you need to perform lookups or comparisons with fixed data.
7. Can nested dictionaries cause the “unhashable type: ‘dict'” error?
Yes, if you try to use a nested dictionary as a key or set element, you’ll encounter this error. Even if the outer structure is hashable, any mutable elements inside it (like dictionaries) will trigger the error.
8. What other data types are unhashable in Python?
Aside from dictionaries, other mutable types like lists and sets are also unhashable. These types cannot be used as dictionary keys or set elements unless converted to immutable types like tuples or frozensets.
9. Why does Python require dictionary keys to be hashable?
Python dictionaries use a hashing mechanism to efficiently store and retrieve data. Hashable keys ensure that the key-value pairs in the dictionary remain consistent and accessible through their unique hash values.
10. How do I know if an object is hashable in Python?
You can check if an object is hashable by using the built-in hash()
function. If the object is hashable, the function will return its hash value. If it is unhashable, Python will raise a TypeError
.
Example:
try:
hash(my_object)
except TypeError:
print(“This object is unhashable.”) . TypeError: unhashable type: ‘dict’
Read Also: Diggin Cafe Chanakyapuri Nearest Metro Station
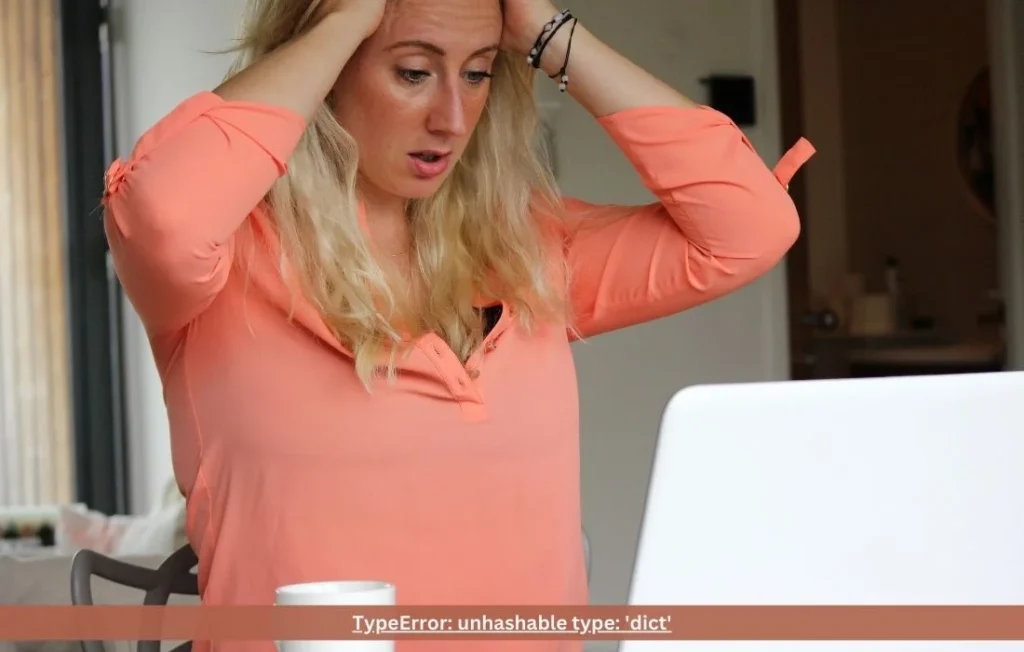
Conclusion
The “TypeError: unhashable type: ‘dict'” is a common error that occurs when a mutable dictionary is used in places where hashable objects are expected, such as keys in dictionaries or elements in sets. Understanding that dictionaries are mutable and therefore unhashable helps you prevent this error by either converting them to immutable types or ensuring you’re using appropriate data structures for your operation. Once you grasp the concept of hashable and unhashable types in Python, this error becomes much easier to resolve and avoid.