Syntax Error: Eol While Scanning String Literal
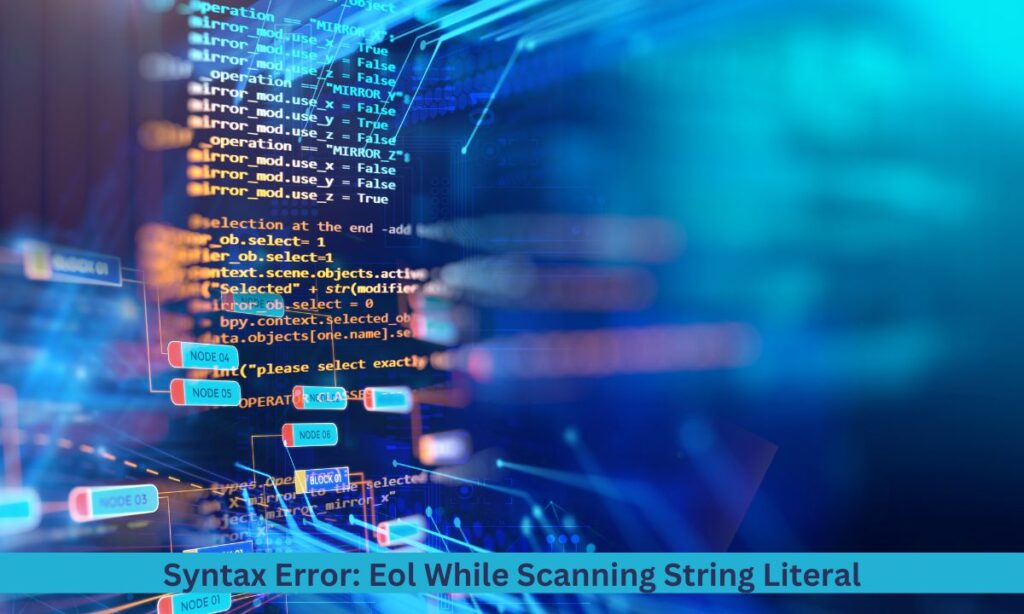
syntaxerror: eol while scanning string literal
When diving into coding, especially with languages like Python, encountering errors is a part of the journey. One common issue developers face is the “syntaxerror: eol while scanning string literal,” This might sound technical and intimidating, but understanding it can save you from hours of frustration. In this article, we’ll break down what this error means, why it occurs, and how to resolve it effectively.
What is the Error “syntaxerror: eol while scanning string literal,
Definition of EOL
EOL stands for “End of Line.” In programming, it refers to the point where a line of code finishes. When the Python interpreter encounters an unexpected end of a line while it’s still scanning a string literal, it raises this specific syntax error.
Explanation of String Literal in Python
A string literal is a sequence of characters enclosed in quotes. In Python, you can use single quotes (‘ ‘), double quotes (” “), or triple quotes (”’ ”’ or “”” “””). These quotes define where the string starts and ends. For instance:
Read Also: Type Error: Only Integer Scalar Arrays Can Be Converted To a Scalar Index
message = "Hello, world!"
Description of the Error
The “SyntaxError: EOL while scanning string literal” occurs when Python expects a closing quote to finish a string literal but instead encounters the end of the line. Essentially, the interpreter is left hanging because it doesn’t find the expected closing quote.
Reasons for the “SyntaxError: EOL While Scanning String Literal”
Missing Closing Quotes
The most common cause of this error is forgetting to close a string with the appropriate quote. For example:
message = "Hello, world
In the example above, the closing quote is missing, leading to the error.
Improper String Termination
Improperly terminating a string can also trigger this error. Consider the following:
Read Also: Error: SRC Refspec Master Does Not Match Any
message = "Hello, world!
Here, the string is started with a quote but not properly closed.
Unescaped Special Characters
Special characters within a string might cause confusion if not handled properly. For instance, if you have a backslash that isn’t part of an escape sequence:
path = "C:\new_folder
Python interprets the backslash as an escape character, leading to unexpected results.
Incorrect String Continuation
If you’re breaking a string across multiple lines without proper continuation:
message = "Hello, world
this is a new line"
Python doesn’t know how to handle the line break within the string literal.
Issues Caused by the “SyntaxError: EOL While Scanning String Literal”
Impact on Code Execution
A syntax error halts the execution of your program. This means that if there is a “SyntaxError: EOL while scanning string literal”, your code won’t run at all until the error is resolved.
Read Also: Get_Ready_Bell:Client_Pulse: Unlocking Customer Insights
Debugging Challenges
This error can sometimes be tricky to debug, especially in large files or complex codebases. You might have to carefully examine each line to find where the mistake lies.
Confusion for Beginners
For those new to programming, understanding and fixing this error can be confusing. It’s important to learn how to identify and correct such issues to avoid frustration.
Troubleshooting the “SyntaxError: EOL While Scanning String Literal”
Identifying the Error Location
Check the error message carefully. It usually provides a line number where the error occurred. Look at the line and possibly the preceding lines to find where the string literal is not properly closed.
Fixing Missing Quotes
Ensure that every string literal has both a starting and an ending quote. For example:
message = "Hello, world!"
Handling Special Characters
If you use special characters like backslashes, ensure they are properly escaped:
path = "C:\\new_folder"
Correcting String Continuation Issues
For multi-line strings, use triple quotes or ensure proper continuation:
message = """Hello, world
this is a new line"""
Code Examples and Solutions
Example 1: Missing Closing Quotes
- Incorrect Code:
text = "Hello, Python
- Corrected Code:
text = "Hello, Python"
Example 2: Improper String Termination
- Incorrect Code:
text = "Python programming
- Corrected Code:
text = "Python programming"
Example 3: Unescaped Special Characters
- Incorrect Code:
file_path = "C:\documents\file.txt"
- Corrected Code:
file_path = "C:\\documents\\file.txt"
Example 4: Incorrect String Continuation
- Incorrect Code:
message = "Hello, world
Welcome to Python!"
- Corrected Code:
message = """Hello, world
Welcome to Python!"""
Best Practices to Avoid Syntax Errors
Consistent Coding Style
Adopt a consistent coding style to reduce the chances of syntax errors. Follow conventions for string delimiters and line breaks.
Read Also: Java.Lang. out of Memory Error: Java Heap Space
Use of IDEs and Linters
Integrated Development Environments (IDEs) and linters can help spot syntax errors before running the code. They provide real-time feedback and suggestions.
Thorough Testing and Debugging
Regularly test and debug your code. Writing and running unit tests can help identify issues early and ensure your code runs as expected.
FAQs
Q. What does EOL stand for in programming?
A. EOL stands for “End of Line.” It indicates where a line of code finishes in the source file.
Q. How can I prevent syntax errors in my code?
A. To prevent syntax errors, follow consistent coding practices, use IDEs with syntax highlighting, and thoroughly test your code.
Q. What tools can help identify syntax errors?
A. IDEs like PyCharm or Visual Studio Code, along with linters like pylint, can help identify syntax errors as you code.
Q. Can this error occur in languages other than Python?
A. Yes, similar errors can occur in other programming languages, though the specifics might vary. The concept of missing end-of-line characters applies broadly.
Q. How do I handle complex strings in Python?
A. For complex strings, use triple quotes to span multiple lines, and ensure proper escaping of special characters.
Conclusion
The “SyntaxError: EOL while scanning string literal” is a common error that occurs when Python encounters an incomplete string literal. By understanding the causes and solutions, you can quickly resolve this issue and improve your coding skills. Remember to always check for missing quotes, handle special characters properly, and use best practices to avoid syntax errors in your code.